Understanding Duplicate Characters in a String in C
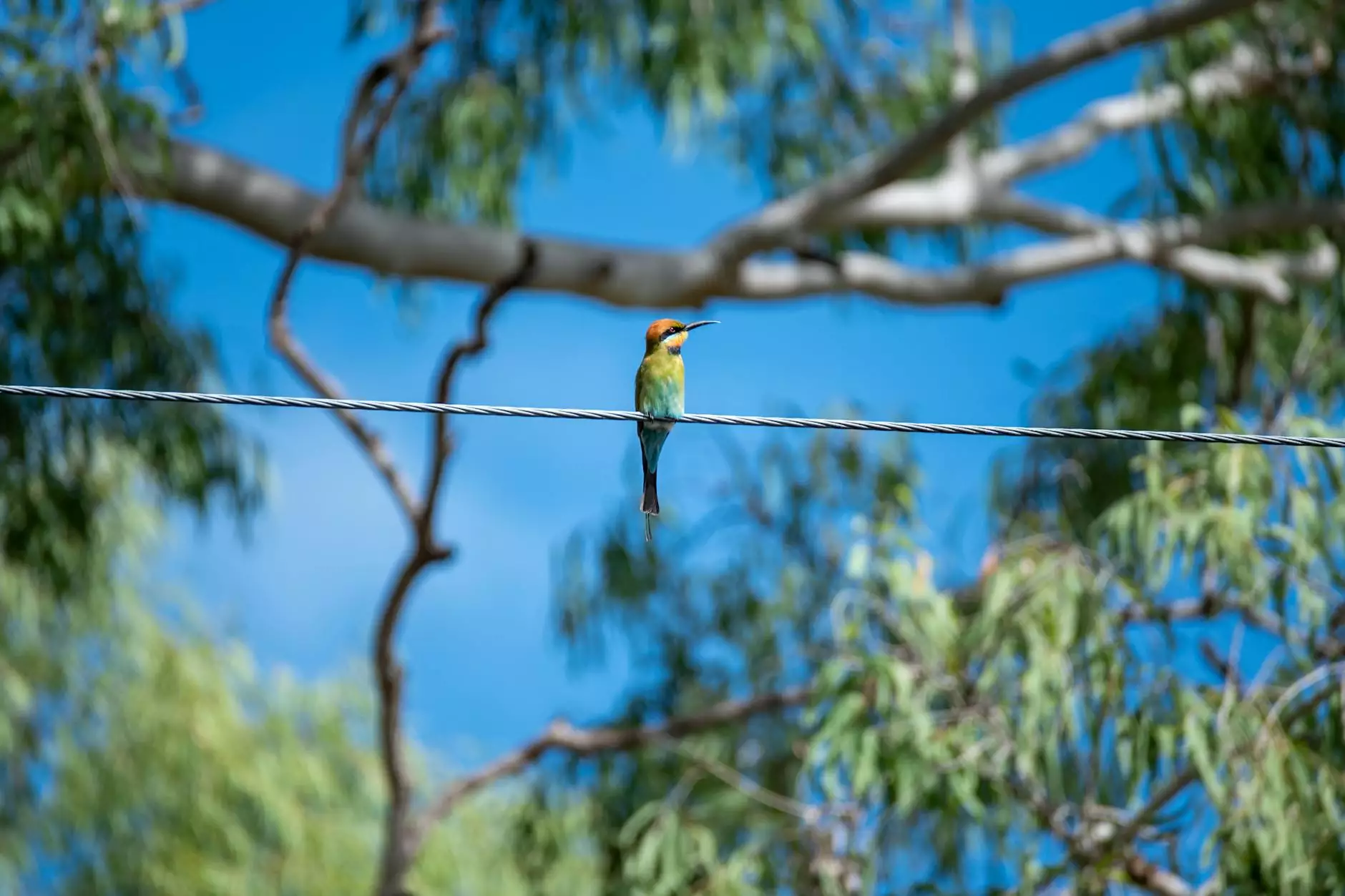
In the realm of programming, one of the common challenges developers encounter is managing and manipulating strings. When dealing with strings in the C programming language, identifying and handling duplicate characters can become essential for various applications, including data analysis, text processing, and software development. In this article, we will delve deeply into the techniques for effectively identifying and managing duplicate characters in a string in C.
Why is it Important to Identify Duplicate Characters?
Detecting duplicate characters in a string is crucial for several reasons:
- Data Validation: Ensuring data integrity by removing or managing duplicates before processing.
- Improved Efficiency: Efficient algorithms can optimize search and storage resources.
- Business Logic: Unique representations may be required for certain functionalities in software applications.
Basic Concepts of Strings in C
Before diving into duplication detection, it is essential to understand how strings work in the C programming language. Unlike higher-level languages, C does not have a specific string data type. Instead, strings are represented as arrays of characters terminated by a null character ('\0').
For example:
char str[] = "Hello, World!";The above code defines a string containing 13 characters, including the null terminator.
Methods for Identifying Duplicate Characters in C
There are several methods to identify duplicate characters in a string using C. Let us explore a few effective approaches.
1. Using Nested Loops
The simplest method involves using nested loops to compare each character in the string with every other character. While straightforward, this approach has a time complexity of O(n2), making it inefficient for long strings.
Here is a sample code snippet:
#include #include void findDuplicates(char *str) { int length = strlen(str); printf("Duplicate characters in the string:\n"); for(int i = 0; iduplicate characters in a string in c 0934225077