How to Create a Chat App in Android: A Comprehensive Guide
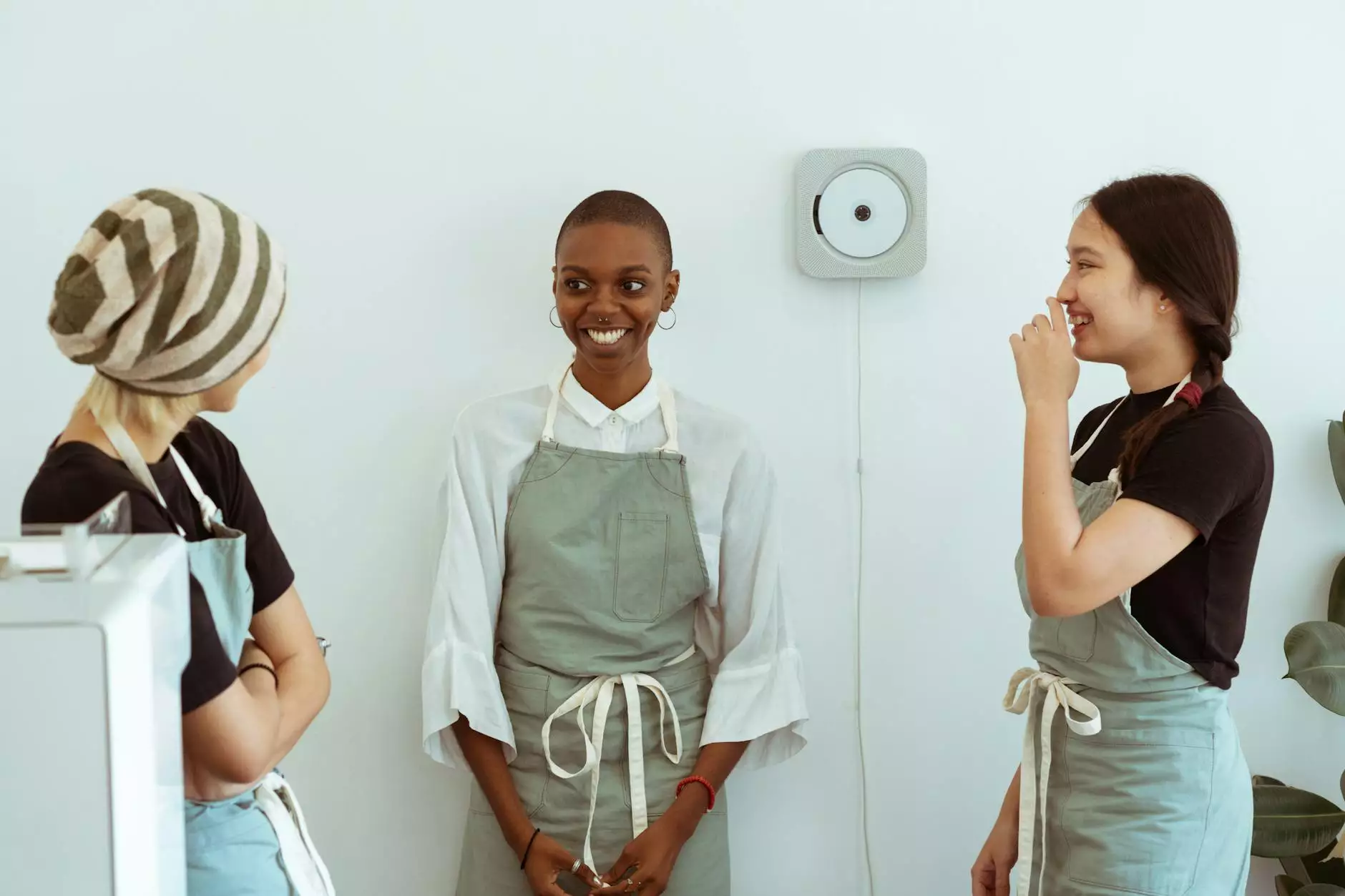
In the world of mobile communication, chat applications have become one of the most essential tools for interaction. From text messages to multimedia sharing, creating a chat app can offer immense value to users. If you are looking to dive into the software development realm and learn how to create a chat app in Android, you’re in the right place!
Understanding the Basics of Chat Applications
Before jumping into the technical details, it’s vital to understand the architecture and functionality of chat applications:
- Real-time Messaging: Chat apps utilize real-time messaging protocols to ensure users receive messages instantly.
- User Accounts: Most chat applications require user registration and account management.
- Media Sharing: Users often want to share images, videos, and other media files in addition to text.
- Group Chats: Enable multiple users to communicate simultaneously.
- Push Notifications: Keeps users informed of new messages even when the app is not in use.
Selecting the Right Tools
To build a functional chat app in Android, you will need to choose the right set of tools and technologies. Here are some of the essentials:
- Programming Language: Java or Kotlin (Kotlin is now the preferred choice for Android development).
- Development Environment: Android Studio is the official IDE for Android development.
- Backend Services: Firebase or AWS can be used to manage user data and real-time messaging.
- User Interface Design: Utilize Android’s Layout Editor to create an intuitive user interface.
Step-by-Step Guide to Creating a Chat App in Android
Step 1: Set Up Your Development Environment
Start by downloading and installing Android Studio. It comes equipped with everything you need to start developing Android applications. Configure your SDK and create a new project.
Step 2: Design the User Interface
The user interface is critical to the success of your chat app. Consider the following:
- Login/Registration Screen: Allow users to create accounts or login.
- Chat Interface: Design a simple chat interface where messages can be sent and displayed.
- User Profile: Let users customize their profiles, adding a profile image or status message.
Step 3: Implement Real-Time Messaging
Choose a backend service that offers real-time database capabilities. For instance, Firebase Realtime Database allows you to sync data across all clients in real-time.
FirebaseDatabase database = FirebaseDatabase.getInstance(); DatabaseReference myRef = database.getReference("messages");With the above code snippet, you can interact with your Firebase database. Ensure you have implemented security rules to protect user data effectively.
Step 4: User Authentication
Using Firebase Authentication, you can integrate email/password login or social media logins. Here’s how:
// Example for email/password authentication FirebaseAuth mAuth = FirebaseAuth.getInstance(); mAuth.createUserWithEmailAndPassword(email, password) .addOnCompleteListener(this, task -> { if (task.isSuccessful()) { // Sign in success } else { // If sign in fails } });Step 5: Sending and Receiving Messages
Implement functionalities for sending and retrieving messages from your database:
myRef.push().setValue(new Message(messageText, currentUserId));This code snippet demonstrates how to add a new message to your Firebase database. Make sure to create a Message class that defines the structure of a message in your app.
Step 6: Group Chats and Multimedia Features
For a more advanced chat app, implement group chats. You can achieve this by creating different chat rooms in your database structure:
DatabaseReference groupChatRef = database.getReference("groupChats").child(groupId); groupChatRef.push().setValue(new Message(messageText, currentUserId));For multimedia messages, you can follow similar steps but include file upload functionality using Firebase Storage.
Step 7: Push Notifications
Integrating push notifications will enhance user experience and keep users informed of new messages:
- Use Firebase Cloud Messaging (FCM) to send notifications.
- Implement notification listeners in your application.
Step 8: Testing Your Application
Once you have completed the development, it’s crucial to test your application:
- User Testing: Gather feedback from potential users.
- Beta Testing: Release a beta version for more extensive testing.
- Performance Testing: Ensure your app handles scaling effectively with multiple users.
Step 9: Launch Your Chat App
After thorough testing and improvements based on user feedback, it’s time to launch your application. Consider using:
- Google Play Store: Most common platform for Android apps.
- Promotion Strategies: Use social media and influencer marketing to promote your app.
Conclusion
Creating a chat app in Android can be a rewarding experience, both for you as a developer and for your users. By following the steps outlined above, you can build a robust and feature-rich application that serves the needs of modern communication.
Remember, the key to success lies in understanding your users and continuously improving your application based on their feedback. Embrace new technologies and trends to keep your chat app relevant in an ever-evolving digital landscape.
Future Improvements and Features
As technology advances, you can enhance your chat application with features such as:
- End-to-End Encryption: Enhance security by implementing encryption for messages.
- Voice and Video Calling: Integrate VoIP services for voice and video communication.
- Artificial Intelligence: Implement chatbots to assist users with inquiries and enhance user engagement.
By focusing on innovation and user needs, your chat app can not only thrive but also become a preferred platform for communication.